Java's version 1.5 introduced more of features to help to deal with multi-threading programs. One of these features is a class which allows a main thread to wait another threads before continue. The name of this class is CountDownLatch.
Data Engineering Design Patterns
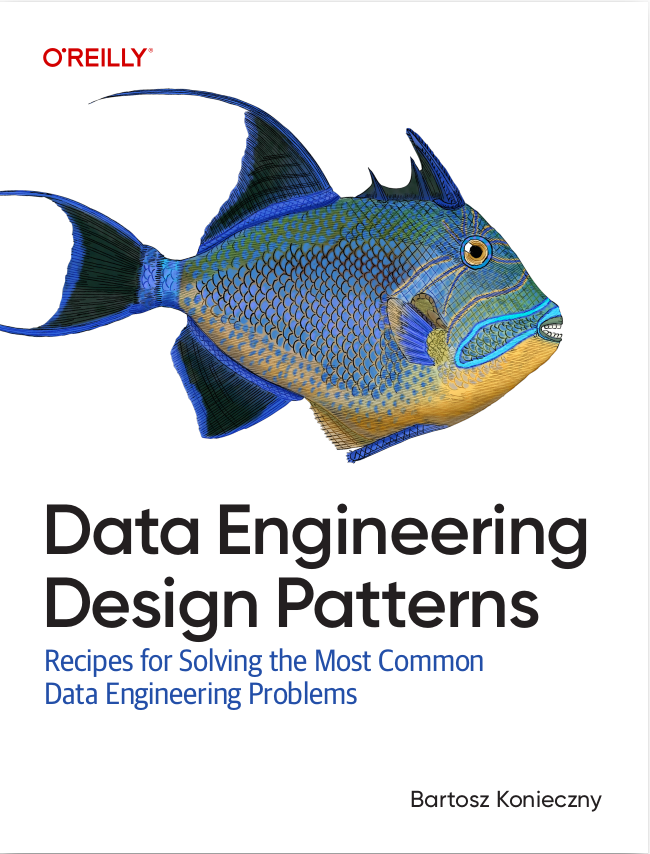
Looking for a book that defines and solves most common data engineering problems? I wrote
one on that topic! You can read it online
on the O'Reilly platform,
or get a print copy on Amazon.
I also help solve your data engineering problems 👉 contact@waitingforcode.com 📩
This article will cover the CountDownLatch concept. In its first part, we'll see the meaning of this concept. After that, a real world use will be translated into Java class.
What is CountDownLatch in Java ?
When you were child, you liked to play football with your friends, right ? But to play a football, you needed at least 4 players, 2 for each small team. You were going to invite your first friend. He accepted. After, you were going to see the second and the third. They accepted too. So, you were 4 and you were able to play. Imagine now that "football playing" is like a main thread in Java application and 4 players (you and your 3 friends) are thread that the main thread needs to work. One by one, you and your friends, are accepted to play, and at the end nobody rests to be invited. Football playing can start now.
CountDownLatch is exactly like the activity explained in previous paragraph. One main thread (football playing) are waiting for N another threads (4 players) before starting its work (match playing). More precisely, CountDownLatch is a class that single constructor takes an int parameter CountDownLatch(int count). This parameter means the number of threads to wait. Now we can start these threads and invoke await() method of CountDownLatch instance. This invocation causes that the main thread will wait N another threads before resumes its job.
After the end of execution, each thread that the main thread must wait, calls CountDownLatch's countDown() method. This call decreases the number N taken in constructor. When this number reaches 0, the main thread's job resumes.
CountDownLatch example
Not complicated, isn't it ? Let's translate the real world example from the previous paragraph into Java's world.
import java.util.concurrent.CountDownLatch; public class Tester{ public static void main(String[] args){ CountDownLatch latch=new CountDownLatch(4); Thread playerOne=new Thread(new Friend("Friend #1", latch)); Thread playerTwo=new Thread(new Friend("Friend #2", latch)); Thread playerThree=new Thread(new Friend("Friend #3", latch)); Thread playerFour=new Thread(new Friend("Friend #4", latch)); playerOne.start(); playerTwo.start(); playerThree.start(); playerFour.start(); try{ latch.await(); } catch(Exception e){ e.printStackTrace(); } System.out.println("We are 4. Let's start to play !"); } }
As you can observe, this code is compact and very clear. At the begin, we initialize new instance of CountDownLatch class. After that we do the same with our 4 friends. We consider that these instances are the threads. And, the most important place, the invocation of latch.await() which signals that before continue, the main thread has to wait the end of execution of 4 another threads. The await() method can be considered here as a kind of barrier that can't be cross until CountDownLatch count parameter is not equal to 0.
import java.util.Random; import java.util.concurrent.CountDownLatch; public class Friend implements Runnable{ private CountDownLatch latch; private String name; public Friend(String name, CountDownLatch latch){ this.name=name; this.latch=latch; System.out.println("We are inviting "+name+" to play"); } @Override public void run(){ Random r=new Random(); try{ Thread.sleep(r.nextInt(5000)); } catch(Exception e){ e.printStackTrace(); } this.latch.countDown(); System.out.println("OK, I'm ready to play - said "+this); } @Override public String toString(){ return "Friend {"+this.name+"}"; } }
This class implements Runnable to be able to be launched in Thread. We observe that after every call of sleep(), the class notifies CountDownLatch instance about the completed job. CountDownLatch gets this notification and decreases the number of threads to wait, until reaching 0.
CountDownLatch is a class that we can use to facilitate the synchronization between threads. Once all secondary threads complete their job, the main thread will resume its task. But remember that it's your secondary thread that must decrement CountDownLatch parameter with number of task to accomplish (countDown()).
Consulting
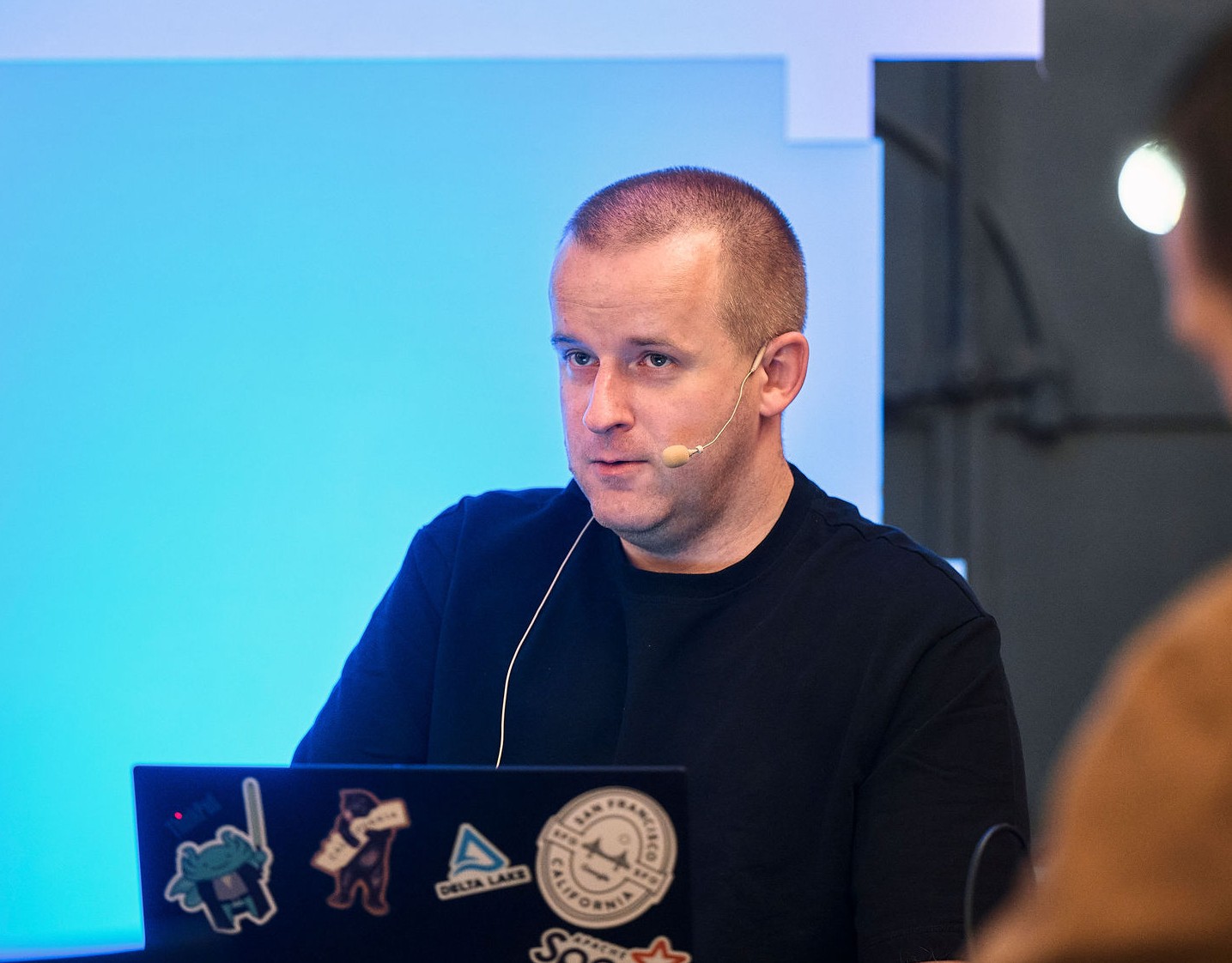
With nearly 16 years of experience, including 8 as data engineer, I offer expert consulting to design and optimize scalable data solutions.
As an O’Reilly author, Data+AI Summit speaker, and blogger, I bring cutting-edge insights to modernize infrastructure, build robust pipelines, and
drive data-driven decision-making. Let's transform your data challenges into opportunities—reach out to elevate your data engineering game today!
👉 contact@waitingforcode.com
đź”— past projects