Generational garbage collection is one of strategies used to automatically remove useless objects from memory. JVM has 2 categories of generations: young and old.
Data Engineering Design Patterns
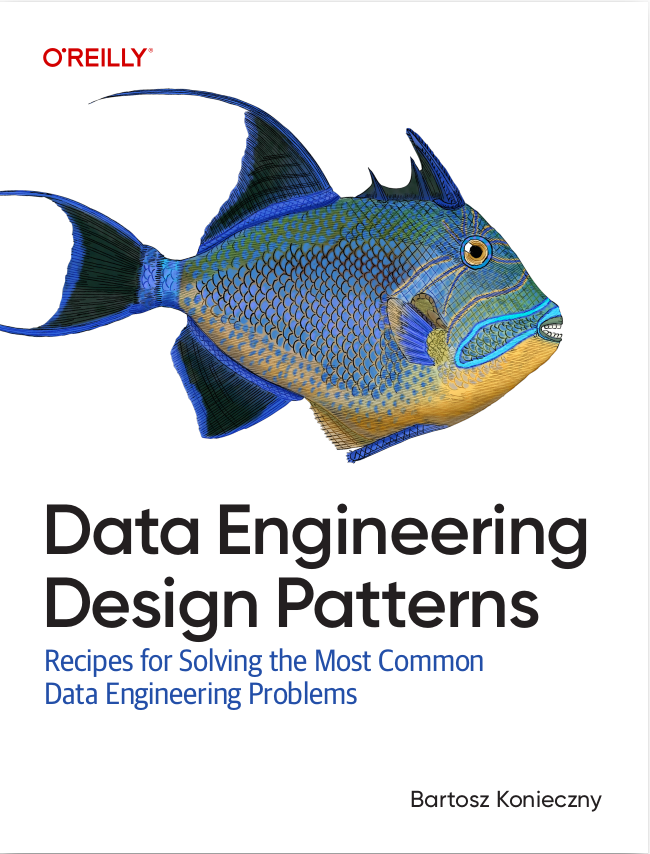
Looking for a book that defines and solves most common data engineering problems? I wrote
one on that topic! You can read it online
on the O'Reilly platform,
or get a print copy on Amazon.
I also help solve your data engineering problems 👉 contact@waitingforcode.com 📩
In this article we'll discover these 2 generations - in 2 first parts. We'll also approach one of concepts related to them, stop-the-world event.
What is young generation ?
Every initialized object goes to JVM heap, and more exactly, to eden space located in young generation. Now, the first garbage collection on this generation occurs. Some of objects aren't reachable anymore, so they are directly removed and remaining free space between them is compacted. The rest of objects, still referenced, moves to a subpart called survivor space. This kind of collection is called minor and it causes a stop-the-world event. However, it influences latency less than the same event triggered from old generation. Minor collection is triggered when, for example, eden space is full and new object can't be created (allocation failure).
The 'age' of objects surviving to collection is increased every time when GC runs. If this age reaches a specific value, related object can be promoted to old generation. Meanwhile, the object continues to be a part of young generation. However, it lives not anymore in eden space but in one of 2 survivor spaces: from or to. One of them is always empty. It means that survived objects from survivor and eden space moves together between from and to spaces. Only when one of moving objects is considered as 'old enough', it's promoted to old generation.
But why one survivor space is always empty ? The principle hidden behind this comes from Copying Garbage Collection. It helps to avoid fragmentation work because it copies all still reachable objects to an empty memory space. If it was a simple removal, GC should in additionally compact memory space, so eliminate holes caused by removed objects. By doing a copy, removal holes are automatically compacted.
What is old generation ?
Old generation is also known as tenured generation, so don't be surprise if you meet this term elsewhere. As young generation keeps young objects, old generation is supposed to keep only old ones. And it's true. But which is the criterion of age classification in Java memory ? This criterion is called tenuring threshold and means the number of times when given objects survived garbage collection. So if one object, placed initially in young generation, survived to 4 last garbage actions, its age is 4. Suppose also that we configured the minimum age of old generations objects to 5. If 4 "survivals" old object survives to another one collection, it's promoted to old generation.
After this short story we can tell that old generation stores objects which are supposed to live very long time (because they survive consecutive young generation collections). The size of old generation is usually bigger than for young generation. Logically, it involves longer collection process. This collection process is called major collection and makes an event called "stop-the-world event". Unlike the same event in minor collection, this one usually takes more time but is executed rarely (old generation size is bigger).
Stop-the-world event
Garbage collections can have small and big impact on the JVM. As you already know, collections with small impact are called minor while with the big one, major. Major collections cause an important stop-the-world (STW). Wut why it's called so ? This names from the fact that everything is interrupted. When this event occurs, all running threads are stopped. Only GC thread is running. When collection process ends, stopped threads restart.
Execution time of major collection will depend on the old generation size. The collection consists on deleting all unreachable objects and compacting space by moving still living objects at the begin of the generation. This collection doesn't occur frequently because old objects are supposed to be long-lived.
Playing with generations
After this introduction, the time is to play with old generation. In this little game, we'll try to observe the behaviour of GC when some of old generation flags are defined. First, we'll try to put all created objects to old generation every time with -XX:+AlwaysTenure parameter. Next, we'll compare execution time with the same code doesn't contain AlwaysTenure flag. That is the code used for tests:
public class OldGenerationRun { public static void main(String[] args) { long expectedEnd = System.currentTimeMillis() + 5000; Map<Integer, List<Integer>> container = new HashMap<>(); for (int j = 0; j < 200_000; j++) { container.put(j, new ArrayList<>()); } int i = 0; while (System.currentTimeMillis() < expectedEnd) { if (i == 20_000) { i = 0; } container.put(i, new ArrayList<>()); for (int j = 0; j < 20_000; j++) { container.get(i).add(j); } i++; } } }
- No flags
- number of garbage collections: 12
- old generation size from 86016kb to 515584kb (~ 6 times growth)
- reasons of GC: allocation failure (9), ergonomics (3)
- allocation failure GC times (in seconds): 0.030, 0.036, 0.057, 0.036, 0.058, 0.06, 0.123, 0.138, 0.155
- ergonomics GC times (in seconds): 1.13, 0.98, 2.63
- young generation almost always fully used when GC (10 of 12 collections times)
- old generation never approached to full occupancy
1/ PSYoungGen total 37888K, used 32768K 2/ PSYoungGen total 37888K, used 37876K 3/ PSYoungGen total 70656K, used 70646K 4/ PSYoungGen total 70656K, used 5093K 5/ PSYoungGen total 70656K, used 65536K 6/ PSYoungGen total 95744K, used 95731K 7/ PSYoungGen total 136192K, used 5116K 8/ PSYoungGen total 136192K, used 131072K 9/ PSYoungGen total 168960K, used 168955K 10/ PSYoungGen total 180736K, used 180724K 11/ PSYoungGen total 239616K, used 239610K 12/ PSYoungGen total 264192K, used 115683K 1/ ParOldGen total 86016K, used 0K 2/ ParOldGen total 86016K, used 41993K 3/ ParOldGen total 86016K, used 78924K 4/ ParOldGen total 180736K, used 83621K 5/ ParOldGen total 180736K, used 107847K 6/ ParOldGen total 180736K, used 148842K 7/ ParOldGen total 304128K, used 153769K 8/ ParOldGen total 304128K, used 163370K 9/ ParOldGen total 304128K, used 205484K 10/ ParOldGen total 304128K, used 229609K 11/ ParOldGen total 304128K, used 272144K 12/ ParOldGen total 515584K, used 304087K 1/ from space 5120K, 0% used [0x00000000d8e00000,0x00000000d8e00000,0x00000000d9300000) to space 5120K, 0% used [0x00000000d8900000,0x00000000d8900000,0x00000000d8e00000) 2/ from space 5120K, 99% used [0x00000000d8900000,0x00000000d8dfd3d0,0x00000000d8e00000) to space 5120K, 0% used [0x00000000dae00000,0x00000000dae00000,0x00000000db300000) 3/ from space 5120K, 99% used [0x00000000dae00000,0x00000000db2fda68,0x00000000db300000) to space 5120K, 0% used [0x00000000da900000,0x00000000da900000,0x00000000dae00000) 4/ from space 5120K, 99% used [0x00000000da900000,0x00000000dadf9500,0x00000000dae00000) to space 5120K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000df300000) 5/ from space 5120K, 0% used [0x00000000da900000,0x00000000da900000,0x00000000dae00000) to space 5120K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000df300000) 6/ from space 5120K, 99% used [0x00000000dee00000,0x00000000df2fcd90,0x00000000df300000) to space 5120K, 0% used [0x00000000de900000,0x00000000de900000,0x00000000dee00000) 7/from space 5120K, 99% used [0x00000000de900000,0x00000000dedff2a8,0x00000000dee00000) to space 49664K, 0% used [0x00000000e1980000,0x00000000e1980000,0x00000000e4a00000) 8/ from space 5120K, 0% used [0x00000000de900000,0x00000000de900000,0x00000000dee00000) to space 49664K, 0% used [0x00000000e1980000,0x00000000e1980000,0x00000000e4a00000) 9/ from space 49664K, 99% used [0x00000000e1980000,0x00000000e49fee90,0x00000000e4a00000) to space 61440K, 0% used [0x00000000ddd80000,0x00000000ddd80000,0x00000000e1980000) 10/ from space 61440K, 99% used [0x00000000ddd80000,0x00000000e197d320,0x00000000e1980000) to space 91136K, 0% used [0x00000000e6b00000,0x00000000e6b00000,0x00000000ec400000) 11/ from space 91136K, 99% used [0x00000000e6b00000,0x00000000ec3fe9d0,0x00000000ec400000) to space 115712K, 0% used [0x00000000dfa00000,0x00000000dfa00000,0x00000000e6b00000) 12/ from space 115712K, 99% used [0x00000000dfa00000,0x00000000e6af8e98,0x00000000e6b00000) to space 150528K, 0% used [0x00000000ebf00000,0x00000000ebf00000,0x00000000f5200000)
- -XX:+AlwaysTenure
- number of garbage collections: 10
- reasons of GC: allocation failure (7), ergonomics (3)
- old generation size growth from 86016kb up to 477184kb (5.5 times)
- survivor's from and to spaces decreased from 5120kb to 512kb
- total young + old generations size before and after: 123904kb and 613376kb (~ 5 times)
- allocation failure GC times (in seconds): 0.055, 0.056, 0.092, 0.063, 0.115, 0.125, 0.113
- ergonomics GC times (in seconds): 1.28, 1.68, 2.77
- old generation resized after the 4th GC
- young generation resized 5 times (every 2nd GC)
1/ PSYoungGen total 37888K, used 32768K [0x00000000d6900000, 0x00000000d9300000, 0x0000000100000000) 2/ PSYoungGen total 37888K, used 32768K [0x00000000d6900000, 0x00000000db300000, 0x0000000100000000) 3/ PSYoungGen total 70656K, used 65536K [0x00000000d6900000, 0x00000000db300000, 0x0000000100000000) 4/ PSYoungGen total 70656K, used 0K [0x00000000d6900000, 0x00000000df300000, 0x0000000100000000) 5/ PSYoungGen total 70656K, used 65536K [0x00000000d6900000, 0x00000000df300000, 0x0000000100000000) 6/ PSYoungGen total 90624K, used 90112K [0x00000000d6900000, 0x00000000dee80000, 0x0000000100000000) 7/ PSYoungGen total 131584K, used 0K [0x00000000d6900000, 0x00000000dee80000, 0x0000000100000000) 8/ PSYoungGen total 131584K, used 126464K [0x00000000d6900000, 0x00000000dee80000, 0x0000000100000000) 9/ PSYoungGen total 136192K, used 135680K [0x00000000d6900000, 0x00000000e0f80000, 0x0000000100000000) 10/ PSYoungGen total 136192K, used 0K [0x00000000d6900000, 0x00000000e2180000, 0x0000000100000000) 1/ ParOldGen total 86016K, used 0K 2/ ParOldGen total 86016K, used 24958K 3/ ParOldGen total 86016K, used 48054K 4/ParOldGen total 86016K, used 84614K 5/ ParOldGen total 176128K, used 84351K 6/ ParOldGen total 176128K, used 113430K 7/ ParOldGen total 176128K, used 154136K 8/ ParOldGen total 291840K, used 153753K 9/ ParOldGen total 291840K, used 209794K 10/ ParOldGen total 291840K, used 271060K 1/ from space 5120K, 0% used [0x00000000d8e00000,0x00000000d8e00000,0x00000000d9300000) to space 5120K, 0% used [0x00000000d8900000,0x00000000d8900000,0x00000000d8e00000) 2/ from space 5120K, 0% used [0x00000000d8900000,0x00000000d8900000,0x00000000d8e00000) to space 5120K, 0% used [0x00000000dae00000,0x00000000dae00000,0x00000000db300000) 3/ from space 5120K, 0% used [0x00000000dae00000,0x00000000dae00000,0x00000000db300000) to space 5120K, 0% used [0x00000000da900000,0x00000000da900000,0x00000000dae00000) 4/ from space 5120K, 0% used [0x00000000da900000,0x00000000da900000,0x00000000dae00000) to space 5120K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000df300000) 5/ from space 5120K, 0% used [0x00000000da900000,0x00000000da900000,0x00000000dae00000) to space 5120K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000df300000) 6/ from space 512K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000dee80000) to space 5120K, 0% used [0x00000000de480000,0x00000000de480000,0x00000000de980000) 7/ from space 5120K, 0% used [0x00000000de480000,0x00000000de480000,0x00000000de980000) to space 512K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000dee80000) 8/ from space 5120K, 0% used [0x00000000de480000,0x00000000de480000,0x00000000de980000) to space 512K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000dee80000) 9/ from space 512K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000dee80000) to space 512K, 0% used [0x00000000ded80000,0x00000000ded80000,0x00000000dee00000) 10/ from space 512K, 0% used [0x00000000ded80000,0x00000000ded80000,0x00000000dee00000) to space 512K, 0% used [0x00000000e2100000,0x00000000e2100000,0x00000000e2180000)
- -XX:+NeverTenure
- number of garbage collections: 12
- reasons of GC: allocation failure (9), ergonomics (3)
- old generation size growth from 86016kb up to 503808kb (5.8 times)
- survivor's from and to spaces increased, from 5120kb to, respectively, 114688kb (from space) and 148992kb (to space)
- total young + old generations size before and after: 123904kb and 764416kb (~ 6.1 times)
- allocation failure GC times (in seconds): 0.033, 0.036, 0.055, 0.036, 0.060, 0.068, 0.124, 0.128, 0.169
- ergonomics GC times (in seconds): 1.00, 1.02, 2.37
- old generation resized 3 times
- young generation resized 8 times
1/ PSYoungGen total 37888K, used 32768K 2/ PSYoungGen total 37888K, used 37874K 3/ PSYoungGen total 70656K, used 70635K 4/ PSYoungGen total 70656K, used 5109K 5/ PSYoungGen total 70656K, used 65536K 6/ PSYoungGen total 95232K, used 95225K 7/ PSYoungGen total 134656K, used 5093K 8/ PSYoungGen total 134656K, used 129536K 9/ PSYoungGen total 167936K, used 167918K 10/ PSYoungGen total 179200K, used 179188K 11/ PSYoungGen total 236032K, used 236027K 12/ PSYoungGen total 260608K, used 114682K 1/ ParOldGen total 86016K, used 0K 2/ ParOldGen total 86016K, used 19391K 3/ ParOldGen total 86016K, used 41991K 4/ ParOldGen total 86016K, used 78811K 5/ ParOldGen total 179200K, used 83539K 6/ ParOldGen total 179200K, used 107737K 7/ ParOldGen total 179200K, used 148430K 8/ ParOldGen total 300032K, used 153389K 9/ ParOldGen total 300032K, used 162055K 10/ ParOldGen total 300032K, used 204179K 11/ ParOldGen total 300032K, used 228417K 12/ ParOldGen total 300032K, used 269734K 1/ from space 5120K, 0% used [0x00000000d8e00000,0x00000000d8e00000,0x00000000d9300000) to space 5120K, 0% used [0x00000000d8900000,0x00000000d8900000,0x00000000d8e00000) 2/ from space 5120K, 99% used [0x00000000d8900000,0x00000000d8dfc980,0x00000000d8e00000) to space 5120K, 0% used [0x00000000dae00000,0x00000000dae00000,0x00000000db300000) 3/ from space 5120K, 99% used [0x00000000dae00000,0x00000000db2fae28,0x00000000db300000) to space 5120K, 0% used [0x00000000da900000,0x00000000da900000,0x00000000dae00000) 4/ from space 5120K, 99% used [0x00000000da900000,0x00000000dadfd5e0,0x00000000dae00000) to space 5120K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000df300000) 5/ from space 5120K, 0% used [0x00000000da900000,0x00000000da900000,0x00000000dae00000) to space 5120K, 0% used [0x00000000dee00000,0x00000000dee00000,0x00000000df300000) 6/ from space 5120K, 99% used [0x00000000dee00000,0x00000000df2fe5c8,0x00000000df300000) to space 5120K, 0% used [0x00000000de900000,0x00000000de900000,0x00000000dee00000) 7/ from space 5120K, 99% used [0x00000000de900000,0x00000000dedf9658,0x00000000dee00000) to space 49664K, 0% used [0x00000000e1800000,0x00000000e1800000,0x00000000e4880000) 8/ from space 5120K, 0% used [0x00000000de900000,0x00000000de900000,0x00000000dee00000) to space 49664K, 0% used [0x00000000e1800000,0x00000000e1800000,0x00000000e4880000) 9/ from space 49664K, 99% used [0x00000000e1800000,0x00000000e487b800,0x00000000e4880000) to space 60928K, 0% used [0x00000000ddc80000,0x00000000ddc80000,0x00000000e1800000) 10/ from space 60928K, 99% used [0x00000000ddc80000,0x00000000e17fd2b8,0x00000000e1800000) to space 90112K, 0% used [0x00000000e6780000,0x00000000e6780000,0x00000000ebf80000) 11/ from space 90112K, 99% used [0x00000000e6780000,0x00000000ebf7ef28,0x00000000ebf80000) to space 114688K, 0% used [0x00000000df780000,0x00000000df780000,0x00000000e6780000) 12/ from space 114688K, 99% used [0x00000000df780000,0x00000000e677e980,0x00000000e6780000) to space 148992K, 0% used [0x00000000eba00000,0x00000000eba00000,0x00000000f4b80000)
- -XX:NewRatio=5
- old generation configured to be 5 times bigger than young one
- number of garbage collections: 16
- reasons of GC: allocation failure (12), ergonomics (4)
- old generation size growth from 107520kb up to 539648kb (~ 5 times)
- survivor's from and to spaces increased, from 2560kb to, respectively, 107008kb (from space) and 113152kb (to space)
- total young + old generations size before and after: 123904kb and 759808kb (~ 6.1 times)
- allocation failure GC times (in seconds): 0.017, 0.019, 0.03, 0.03, 0.05, 0.03, 0.07, 0.09, 0.13, 0.15, 0.13, 0.17
- ergonomics GC times (in seconds): 1,27, 0.82, 1.25, 2.28
- young generation resized 10 times
- old generation resized 4 times - more space allocated at the begin, so no need to reallocate it further
1/ PSYoungGen total 18944K, used 16384K 2/ PSYoungGen total 18944K, used 18914K 3/ PSYoungGen total 35328K, used 35298K 4/ PSYoungGen total 35328K, used 35305K 5/ PSYoungGen total 68096K, used 68069K 6/ PSYoungGen total 68096K, used 2536K 7/ PSYoungGen total 68096K, used 65536K 8/ PSYoungGen total 119296K, used 118976K 9/ PSYoungGen total 158720K, used 38370K 10/ PSYoungGen total 158720K, used 127967K 11/ PSYoungGen total 178176K, used 178155K 12/ PSYoungGen total 188928K, used 70125K 13/ PSYoungGen total 188928K, used 147425K 14/ PSYoungGen total 223744K, used 206456K 15/ PSYoungGen total 232448K, used 232445K 16/ PSYoungGen total 220160K, used 106996K 1/ ParOldGen total 107520K, used 0K 2/ ParOldGen total 107520K, used 10700K 3/ ParOldGen total 107520K, used 22428K 4/ ParOldGen total 107520K, used 45500K 5/ ParOldGen total 107520K, used 67294K 6/ ParOldGen total 107520K, used 96908K 7/ ParOldGen total 161280K, used 99014K 8/ ParOldGen total 161280K, used 99014K 9/ ParOldGen total 161280K, used 130444K 10/ ParOldGen total 248320K, used 161047K 11/ ParOldGen total 248320K, used 163699K 12/ ParOldGen total 248320K, used 206482K 13/ ParOldGen total 369152K, used 247782K 14/ ParOldGen total 369152K, used 248933K 15/ ParOldGen total 369152K, used 279524K 16/ ParOldGen total 369152K, used 336201K 1/ eden space 16384K, 100% used [0x00000000eb480000,0x00000000ec480000,0x00000000ec480000) from space 2560K, 0% used [0x00000000ec700000,0x00000000ec700000,0x00000000ec980000) 2/ eden space 16384K, 100% used [0x00000000eb480000,0x00000000ec480000,0x00000000ec480000) from space 2560K, 98% used [0x00000000ec480000,0x00000000ec6f8a60,0x00000000ec700000) 3/ eden space 32768K, 100% used [0x00000000eb480000,0x00000000ed480000,0x00000000ed480000) from space 2560K, 98% used [0x00000000ed700000,0x00000000ed978990,0x00000000ed980000) 4/ eden space 32768K, 100% used [0x00000000eb480000,0x00000000ed480000,0x00000000ed480000) from space 2560K, 99% used [0x00000000ed480000,0x00000000ed6fa728,0x00000000ed700000) 5/ eden space 65536K, 100% used [0x00000000eb480000,0x00000000ef480000,0x00000000ef480000) from space 2560K, 98% used [0x00000000ef700000,0x00000000ef9794f0,0x00000000ef980000) 6/ eden space 65536K, 0% used [0x00000000eb480000,0x00000000eb480000,0x00000000ef480000) from space 2560K, 99% used [0x00000000ef480000,0x00000000ef6fa3c8,0x00000000ef700000) 7/ from space 2560K, 0% used [0x00000000ef480000,0x00000000ef480000,0x00000000ef700000) to space 36352K, 0% used [0x00000000f5800000,0x00000000f5800000,0x00000000f7b80000) 8/ from space 29696K, 98% used [0x00000000f5800000,0x00000000f74b03d0,0x00000000f7500000) to space 38400K, 0% used [0x00000000f2a00000,0x00000000f2a00000,0x00000000f4f80000) 9/ from space 38400K, 99% used [0x00000000f2a00000,0x00000000f4f78b68,0x00000000f4f80000) to space 59392K, 0% used [0x00000000f6d00000,0x00000000f6d00000,0x00000000fa700000) 10/ from space 38400K, 19% used [0x00000000f2a00000,0x00000000f3177f00,0x00000000f4f80000) to space 59392K, 0% used [0x00000000f6d00000,0x00000000f6d00000,0x00000000fa700000) 11/ from space 59392K, 99% used [0x00000000f6d00000,0x00000000fa6fad60,0x00000000fa700000) to space 70144K, 0% used [0x00000000f2880000,0x00000000f2880000,0x00000000f6d00000) 12/ from space 70144K, 99% used [0x00000000f2880000,0x00000000f6cfb5a0,0x00000000f6d00000) to space 98304K, 0% used [0x00000000fa000000,0x00000000fa000000,0x0000000100000000) 13/ from space 70144K, 40% used [0x00000000f2880000,0x00000000f4478740,0x00000000f6d00000) to space 98304K, 0% used [0x00000000fa000000,0x00000000fa000000,0x0000000100000000) 14/ from space 98304K, 82% used [0x00000000fa000000,0x00000000fef1e190,0x0000000100000000) to space 107008K, 0% used [0x00000000f2f00000,0x00000000f2f00000,0x00000000f9780000) 15/ from space 107008K, 99% used [0x00000000f2f00000,0x00000000f977f630,0x00000000f9780000) to space 107008K, 0% used [0x00000000f9780000,0x00000000f9780000,0x0000000100000000) 16/ from space 107008K, 99% used [0x00000000f9780000,0x00000000ffffd070,0x0000000100000000) to space 113152K, 0% used [0x00000000f2300000,0x00000000f2300000,0x00000000f9180000)
- -XX:SurvivorRatio=11
- total number of GC: 11
- Eden space is 11 times bigger than From and To spaces
- old generation's growth from 86016kb up to 585728kb (6 times)
- fixed size of from and to spaces, always 62-99% occupancy (after the 1st GC) - only one time is equal to 0 because of full GC ergonomics causing heap resizing (from space passed from 3072kb to 51200kb) and promotion objects from survivor space to old generation
- Eden is full 72% of time (8/11 collections). It's empty when all objects are promoted to old generation
- reasons of GC: allocation failure (8 times), ergonomics (3)
- allocation failure GC times (in seconds): 0.032, 0.037, 0.056, 0.063, 0.066, 0.075, 0.153, 0.139
- ergonomics GC times (in seconds): 1.12, 1.12, 2.54
- old generation resized 5 times
1/ eden space 36864K, 100% used [0x00000000d6900000,0x00000000d8d00000,0x00000000d8d00000) 2/ eden space 36864K, 100% used [0x00000000d6900000,0x00000000d8d00000,0x00000000d8d00000) 3/ eden space 73728K, 100% used [0x00000000d6900000,0x00000000db100000,0x00000000db100000) 4/ eden space 73728K, 0% used [0x00000000d6900000,0x00000000d6900000,0x00000000db100000) 5/ eden space 73728K, 100% used [0x00000000d6900000,0x00000000db100000,0x00000000db100000) 6/ eden space 101888K, 100% used [0x00000000d6900000,0x00000000dcc80000,0x00000000dcc80000) 7/ eden space 147456K, 0% used [0x00000000d6900000,0x00000000d6900000,0x00000000df900000) 8/ eden space 147456K, 100% used [0x00000000d6900000,0x00000000df900000,0x00000000df900000) 9/ eden space 146432K, 100% used [0x00000000d6900000,0x00000000df800000,0x00000000df800000) 10/ eden space 146432K, 100% used [0x00000000d6900000,0x00000000df800000,0x00000000df800000) 11/ eden space 211968K, 0% used [0x00000000d6900000,0x00000000d6900000,0x00000000e3800000) 1/ from space 3072K, 99% used [0x00000000d8d00000,0x00000000d8ffdbb8,0x00000000d9000000) 2/ from space 3072K, 99% used [0x00000000db400000,0x00000000db6ff768,0x00000000db700000) 3/ from space 3072K, 99% used [0x00000000db100000,0x00000000db3fee08,0x00000000db400000) 4/ from space 3072K, 66% used [0x00000000db100000,0x00000000db2fe9f8,0x00000000db400000) 5/ from space 3072K, 99% used [0x00000000dfc00000,0x00000000dfefcb80,0x00000000dff00000) 6/ from space 3072K, 99% used [0x00000000df900000,0x00000000dfbfdf30,0x00000000dfc00000) 7/ from space 3072K, 0% used [0x00000000df900000,0x00000000df900000,0x00000000dfc00000) [Full GC (Ergonomics) [PSYoungGen: 3063K->0K(150528K)] [ParOldGen: 166792K->169686K(335872K)] 169855K->169686K(486400K), [Metaspace: 3137K->3137K(1056768K)], 1,1295769 secs] [Times: user=3,85 sys=0,04, real=1,13 secs] 8/ from space 51200K, 99% used [0x00000000e2b00000,0x00000000e5cfddc0,0x00000000e5d00000) 9/ from space 52224K, 99% used [0x00000000df800000,0x00000000e2afba10,0x00000000e2b00000) 10/ from space 52224K, 99% used [0x00000000e6b00000,0x00000000e9df8380,0x00000000e9e00000) 11/ from space 52224K, 62% used [0x00000000e6b00000,0x00000000e8ae63d8,0x00000000e9e00000) 1/ ParOldGen total 86016K, used 24143K [0x0000000083a00000, 0x0000000088e00000, 0x00000000d6900000) object space 86016K, 28% used [0x0000000083a00000,0x0000000085193f38,0x0000000088e00000) 2/ ParOldGen total 86016K, used 49558K [0x0000000083a00000, 0x0000000088e00000, 0x00000000d6900000) object space 86016K, 57% used [0x0000000083a00000,0x0000000086a65938,0x0000000088e00000) 3/ ParOldGen total 89088K, used 88127K [0x0000000083a00000, 0x0000000089100000, 0x00000000d6900000) object space 89088K, 98% used [0x0000000083a00000,0x000000008900ffb8,0x0000000089100000) 4/ ParOldGen total 193024K, used 88742K [0x0000000083a00000, 0x000000008f680000, 0x00000000d6900000) object space 193024K, 45% used [0x0000000083a00000,0x00000000890a9b68,0x000000008f680000) 5/ ParOldGen total 193024K, used 120734K [0x0000000083a00000, 0x000000008f680000, 0x00000000d6900000) object space 193024K, 62% used [0x0000000083a00000,0x000000008afe79f8,0x000000008f680000) 6/ ParOldGen total 193024K, used 166792K [0x0000000083a00000, 0x000000008f680000, 0x00000000d6900000) object space 193024K, 86% used [0x0000000083a00000,0x000000008dce20b8,0x000000008f680000) 7/ ParOldGen total 335872K, used 169686K [0x0000000083a00000, 0x0000000098200000, 0x00000000d6900000) object space 335872K, 50% used [0x0000000083a00000,0x000000008dfb58a8,0x0000000098200000) 8/ ParOldGen total 335872K, used 184965K [0x0000000083a00000, 0x0000000098200000, 0x00000000d6900000) object space 335872K, 55% used [0x0000000083a00000,0x000000008eea15d8,0x0000000098200000) 9/ ParOldGen total 335872K, used 250106K [0x0000000083a00000, 0x0000000098200000, 0x00000000d6900000) object space 335872K, 74% used [0x0000000083a00000,0x0000000092e3eb08,0x0000000098200000) 10/ ParOldGen total 335872K, used 316228K [0x0000000083a00000, 0x0000000098200000, 0x00000000d6900000) object space 335872K, 94% used [0x0000000083a00000,0x0000000096ed1318,0x0000000098200000) 11/ ParOldGen total 585728K, used 335443K [0x0000000083a00000, 0x00000000a7600000, 0x00000000d6900000) object space 585728K, 57% used [0x0000000083a00000,0x0000000098194d50,0x00000000a7600000) Heap PSYoungGen total 264192K, used 41152K [0x00000000d6900000, 0x00000000ea800000, 0x0000000100000000) eden space 211968K, 4% used [0x00000000d6900000,0x00000000d7149ee8,0x00000000e3800000) from space 52224K, 62% used [0x00000000e6b00000,0x00000000e8ae63d8,0x00000000e9e00000) to space 52224K, 0% used [0x00000000e3800000,0x00000000e3800000,0x00000000e6b00000) ParOldGen total 585728K, used 335443K [0x0000000083a00000, 0x00000000a7600000, 0x00000000d6900000) object space 585728K, 57% used [0x0000000083a00000,0x0000000098194d50,0x00000000a7600000) Metaspace used 3143K, capacity 4494K, committed 4864K, reserved 1056768K class space used 336K, capacity 386K, committed 512K, reserved 1048576K
To resume the experiment, we can freely tell that JVM heap is always adapted to existent situation. For example, in the case of -XX:+AlwaysTenure flag we saw that unused survivor spaces decreased 10 times. In the other side, old generation grew 5 times because it accepted more and more objects. We can also observe that full ergonomic GC takes longer than allocation failure GC. And the time needed to execute ergonomic GC is always bigger. In the case of -XX:NewRatio=5 flag GC took approximately 6 seconds to execute ergonomic GC. Also the number of GC was the biggest in this case (16 against 12, 11 and 10).
This article introduces the idea of generations inside Java memory. The first part describes short-lived objects, placed inside young generation. The second part treats about old generation and objects supposed to live much more longer. The third part explains the meaning of stop-the-world event while the last one shows some tuning options and their influence on sizes of described generations.
Consulting
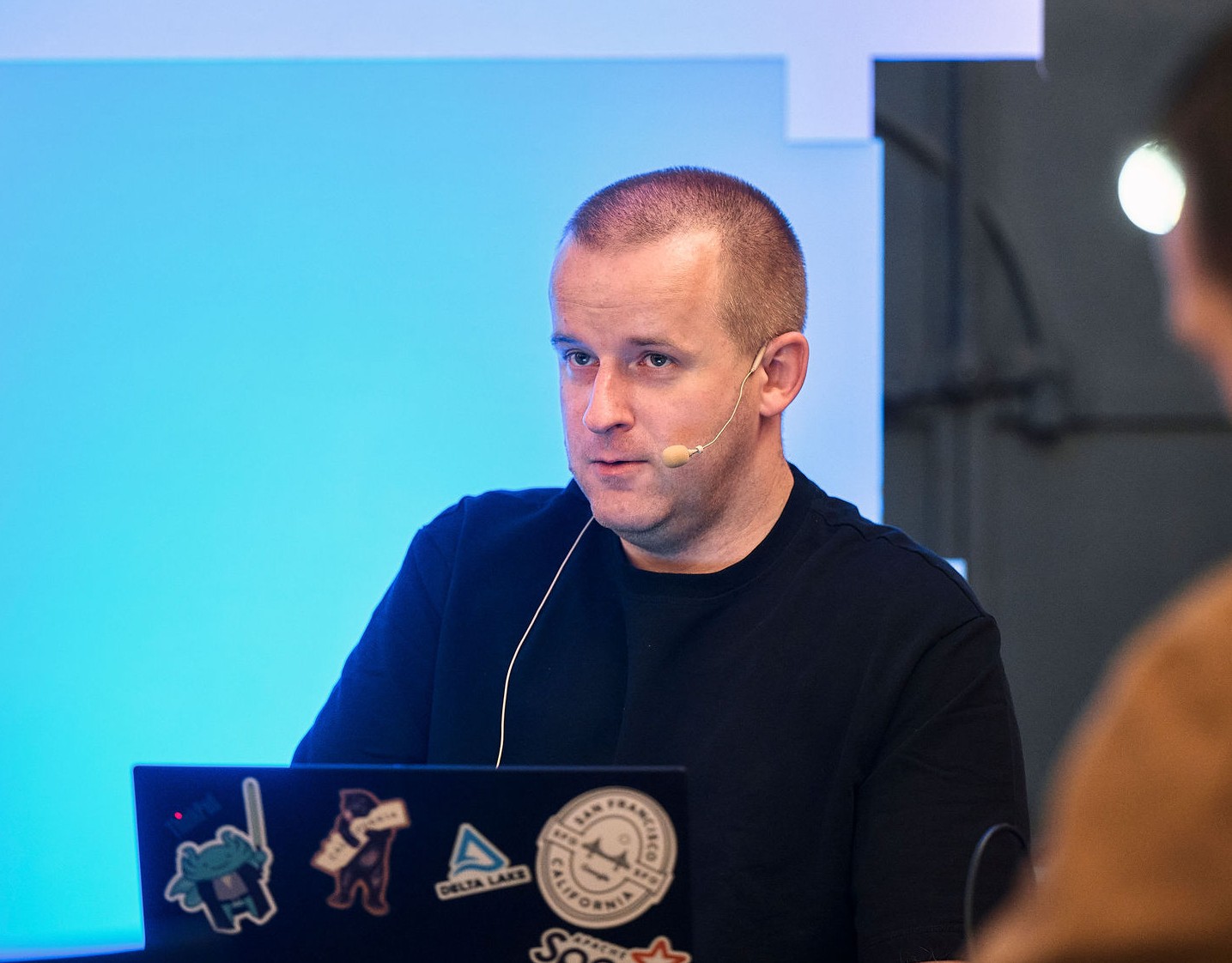
With nearly 16 years of experience, including 8 as data engineer, I offer expert consulting to design and optimize scalable data solutions.
As an O’Reilly author, Data+AI Summit speaker, and blogger, I bring cutting-edge insights to modernize infrastructure, build robust pipelines, and
drive data-driven decision-making. Let's transform your data challenges into opportunities—reach out to elevate your data engineering game today!
👉 contact@waitingforcode.com
đź”— past projects