In the occasion of the post about currying I mentioned shortly the existence of similar concept called partially applied functions. This idea will be explained in this post.
Data Engineering Design Patterns
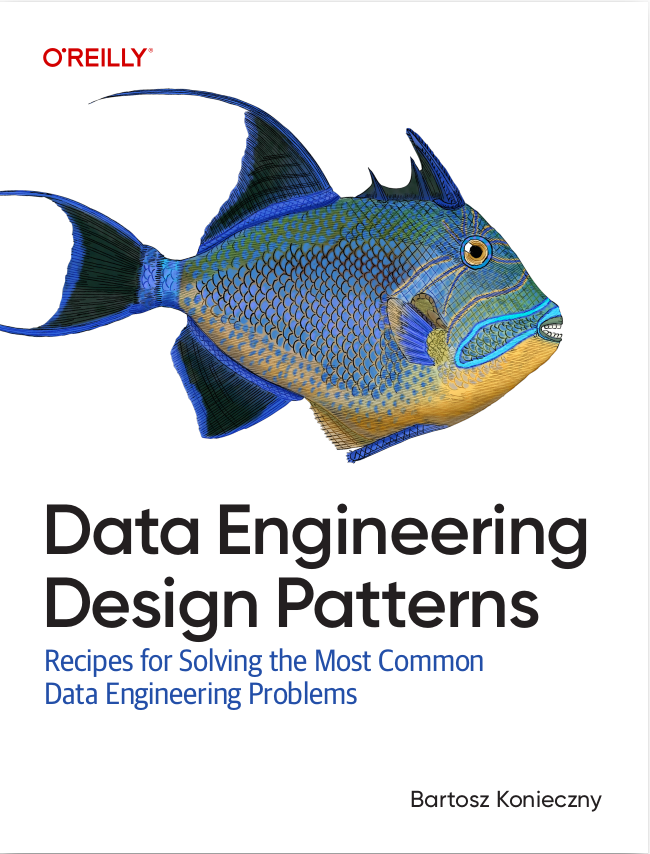
Looking for a book that defines and solves most common data engineering problems? I wrote
one on that topic! You can read it online
on the O'Reilly platform,
or get a print copy on Amazon.
I also help solve your data engineering problems 👉 contact@waitingforcode.com 📩
The first section describes this concept and explains the difference between partially applied functions and currying. The next part contains some code examples showing how the partially applied functions work.
Definition
The partially applied functions concept is very similar to the currying. As currying it fixes some function arguments values. After that step, a new function with reduced number of parameters is constructed. Technically speaking we could tell that the partially applied function reduces the function's arity (number of arguments accepted by the function).
Regarding to the currying, even if basically it also tends to reduce the number of parameters, the partially applied functions are different. As told they reduce the number of undefined parameters and build a new function every time. For the case of currying, it returns a sequence of functions, each one taking a single parameter.
What is then the reason behind the use of partially applied functions ? Among others, it reduces the number of parameters and then allows the creation of more meaningful and shorter functions. An example of that could be an add(int, int) function. From it we could easily build the function incrementByOne(int) = add(1, int) or decrementByOne(int) = add(-1, int). By that the partially applied functions help to enforce functions and logic uniqueness. Thanks to them we can create one or more functions with predefined arguments and thus do not duplicate the logic.
Examples
The partially applied function examples take the case of words concatenation that was already covered in the post about currying in Scala:
describe("partially applied function") { it("should concatenate words with predefined separator") { def concatenate(word1: String, word2: String, separator: String): String = { s"$word1$separator$word2" } val concatenateWithComma: (String, String) => String = concatenate(_, _, ",") val aAndBConcatenatedWithComma = concatenateWithComma("A", "B") aAndBConcatenatedWithComma shouldEqual "A,B" } it("should concatenate words with default argument") { def concatenate(word1: String, word2: String, separator: String = ","): String = { s"$word1$separator$word2" } val concatenateToB: (String) => String = concatenate(_, "B") val aConcatenatedWithBAndDefaultSeparator = concatenateToB("A") aConcatenatedWithBAndDefaultSeparator shouldEqual "A,B" } }
This short post introduced the concept of the partially applied function. This another functional programming concept implemented in Scala can be useful in a lot of places. As explained, it helps to fix some number of function's parameters and thus reduce its arity, a little bit like currying presented in one of previous posts. The second shown how to use this idea in the example of a simple concatenation function.
Consulting
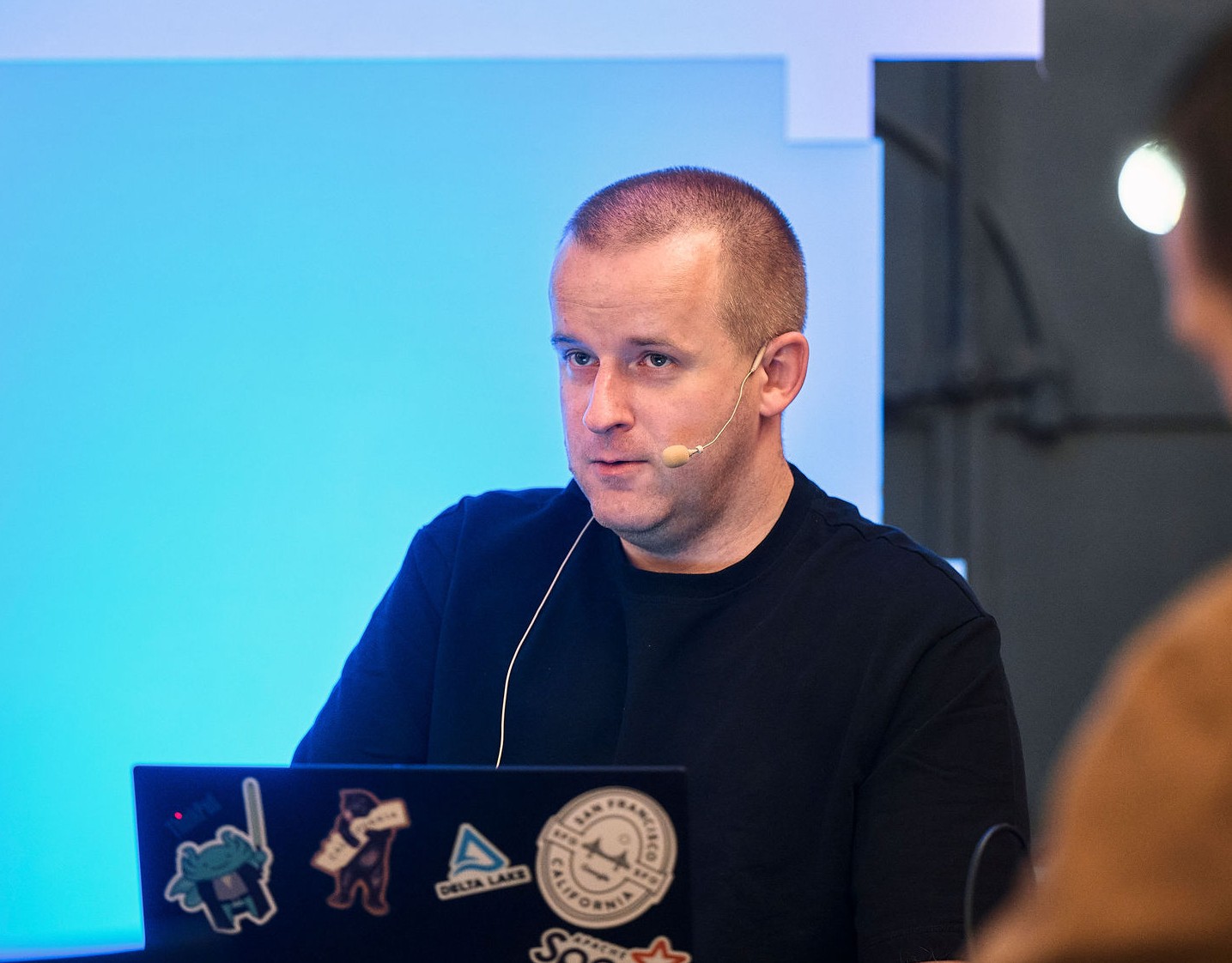
With nearly 16 years of experience, including 8 as data engineer, I offer expert consulting to design and optimize scalable data solutions.
As an O’Reilly author, Data+AI Summit speaker, and blogger, I bring cutting-edge insights to modernize infrastructure, build robust pipelines, and
drive data-driven decision-making. Let's transform your data challenges into opportunities—reach out to elevate your data engineering game today!
👉 contact@waitingforcode.com
đź”— past projects